Android Working with Button
Here, we are going to create two textfields and one button for sum of two numbers. If user clicks one the button, sum of two input values is displayed on the Toast.Drag the component or write the code for UI in activity_main.xml
First of all, drag 2 textfields from the Text Fields palette and one button from the Form Widgets palette as shown in the following figure.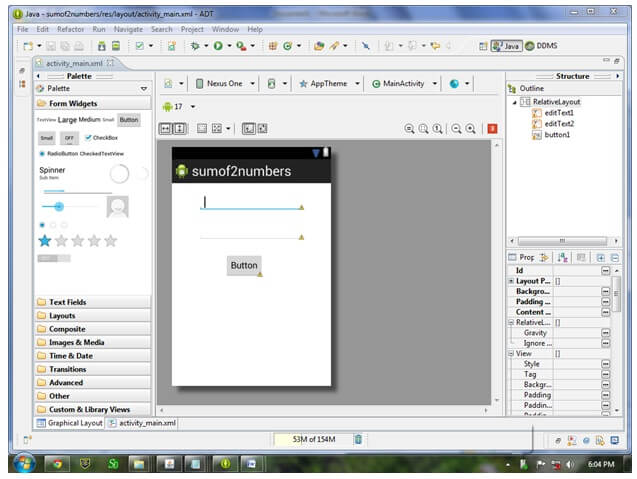
File: activity_main.xml
<RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="24dp"
android:ems="10" />
<EditText
android:id="@+id/editText2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/editText1"
android:layout_below="@+id/editText1"
android:layout_marginTop="34dp"
android:ems="10" >
<requestFocus />
</EditText>
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:text="@string/Button" />
</RelativeLayout>
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="24dp"
android:ems="10" />
<EditText
android:id="@+id/editText2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/editText1"
android:layout_below="@+id/editText1"
android:layout_marginTop="34dp"
android:ems="10" >
<requestFocus />
</EditText>
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:text="@string/Button" />
</RelativeLayout>
Activity class
Now write the code to display the sum of two numbers.
File: MainActivity.java
package com.example.sumof2numbers;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
private EditText edittext1,edittext2;
private Button buttonSum;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
addListenerOnButton();
}
public void addListenerOnButton(){
edittext1=(EditText)findViewById(R.id.editText1);
edittext2=(EditText)findViewById(R.id.editText2);
buttonSum=(Button)findViewById(R.id.button1);
buttonSum.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View view) {
String value1=edittext1.getText().toString();
String value2=edittext2.getText().toString();
int a=Integer.parseInt(value1);
int b=Integer.parseInt(value2);
int sum=a+b;
Toast.makeText(getApplicationContext(),String.valueOf(sum),Toast.LENGTH_LONG).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
private EditText edittext1,edittext2;
private Button buttonSum;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
addListenerOnButton();
}
public void addListenerOnButton(){
edittext1=(EditText)findViewById(R.id.editText1);
edittext2=(EditText)findViewById(R.id.editText2);
buttonSum=(Button)findViewById(R.id.button1);
buttonSum.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View view) {
String value1=edittext1.getText().toString();
String value2=edittext2.getText().toString();
int a=Integer.parseInt(value1);
int b=Integer.parseInt(value2);
int sum=a+b;
Toast.makeText(getApplicationContext(),String.valueOf(sum),Toast.LENGTH_LONG).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
- }
It is developed by adt bundle on android 4.2 using minimum sdk 8 and target sdk 16.
Output:


Android Toggle Button Example
Toggle Button can be used to display checked/unchecked (On/Off) state on the button. It can be used to On/Off Sound, Wifi, Bluetooth etc.ToggleButton class
ToggleButton class provides the facility of creating the toggle button.Constants of ToggleButton class
- android:disabledAlpha The alpha to apply to the indicator when disabled.
- android:textOff The text for the button when it is not checked.
- android:textOn The text for the button when it is checked.
Methods of ToggleButton class
- CharSequence getTextOff() Returns the text when the button is not in the checked state.
- CharSequence getTextOn() Returns the text for when the button is in the checked state.
- void setChecked(boolean checked) Changes the checked state of this button.
Example of ToggleButton
activity_main.xml
Drag two toggle button and one button for the layout. Now the activity_main.xml file will look like this:
File: activity_main.xml
<RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<ToggleButton
android:id="@+id/toggleButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginLeft="60dp"
android:layout_marginTop="18dp"
android:text="ToggleButton1"
android:textOff="Off"
android:textOn="On" />
<ToggleButton
android:id="@+id/toggleButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/toggleButton1"
android:layout_alignBottom="@+id/toggleButton1"
android:layout_marginLeft="44dp"
android:layout_toRightOf="@+id/toggleButton1"
android:text="ToggleButton2"
android:textOff="Off"
android:textOn="On" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/toggleButton2"
android:layout_marginTop="82dp"
android:layout_toRightOf="@+id/toggleButton1"
android:text="submit" />
</RelativeLayout>
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<ToggleButton
android:id="@+id/toggleButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginLeft="60dp"
android:layout_marginTop="18dp"
android:text="ToggleButton1"
android:textOff="Off"
android:textOn="On" />
<ToggleButton
android:id="@+id/toggleButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/toggleButton1"
android:layout_alignBottom="@+id/toggleButton1"
android:layout_marginLeft="44dp"
android:layout_toRightOf="@+id/toggleButton1"
android:text="ToggleButton2"
android:textOff="Off"
android:textOn="On" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/toggleButton2"
android:layout_marginTop="82dp"
android:layout_toRightOf="@+id/toggleButton1"
android:text="submit" />
</RelativeLayout>
Activity class
Let's write the code to check which toggle button is ON/OFF.
File: MainActivity.java
package com.example.togglebutton;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.Toast;
import android.widget.ToggleButton;
public class MainActivity extends Activity {
private ToggleButton toggleButton1, toggleButton2;
private Button buttonSubmit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
addListenerOnButtonClick();
}
public void addListenerOnButtonClick(){
//Getting the ToggleButton and Button instance from the layout xml file
toggleButton1=(ToggleButton)findViewById(R.id.toggleButton1);
toggleButton2=(ToggleButton)findViewById(R.id.toggleButton2);
buttonSubmit=(Button)findViewById(R.id.button1);
//Performing action on button click
buttonSubmit.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View view) {
StringBuilder result = new StringBuilder();
result.append("ToggleButton1 : ").append(toggleButton1.getText());
result.append("\nToggleButton2 : ").append(toggleButton2.getText());
//Displaying the message in toast
Toast.makeText(getApplicationContext(), result.toString(),Toast.LENGTH_LONG).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.Toast;
import android.widget.ToggleButton;
public class MainActivity extends Activity {
private ToggleButton toggleButton1, toggleButton2;
private Button buttonSubmit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
addListenerOnButtonClick();
}
public void addListenerOnButtonClick(){
//Getting the ToggleButton and Button instance from the layout xml file
toggleButton1=(ToggleButton)findViewById(R.id.toggleButton1);
toggleButton2=(ToggleButton)findViewById(R.id.toggleButton2);
buttonSubmit=(Button)findViewById(R.id.button1);
//Performing action on button click
buttonSubmit.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View view) {
StringBuilder result = new StringBuilder();
result.append("ToggleButton1 : ").append(toggleButton1.getText());
result.append("\nToggleButton2 : ").append(toggleButton2.getText());
//Displaying the message in toast
Toast.makeText(getApplicationContext(), result.toString(),Toast.LENGTH_LONG).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Output:

